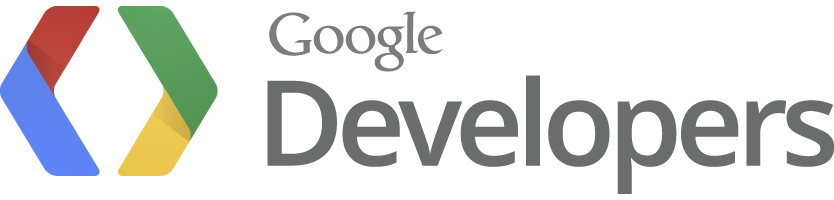
<style> div { background-image: linear-gradient(left top, red 0%, orange 16%, yellow 33%, green 50%, blue 67%, indigo 84%, violet 100%); transform: rotate(20deg); } </style>
<video autoplay width="600" height="480" src=""></video> <canvas width="600" height="480" style="display:none;" ></canvas> <img src="" width="100" height="80" ></img>
navigator.getUserMedia = navigator.webkitGetUserMedia; window.URL = window.webkitURL; function switchOnCamera() { navigator.getUserMedia({video: true;}, successFn, failureFn); } function successFn(localMediaStream) { var video = document.querySelector("video"); video.src = window.URL.createObjectURL(localMediaStream); }
video { -webkit-filter: grayscale(0.4) sepia(0.6) saturate(2.3) hue-rotate(90deg) invert(0.1) opacity(1) brightness(0) contrast(1) blur(0) drop-shadow(10px 10px 10px #008888); }
function takePhoto() { var canvas = document.querySelector('canvas'); var ctx = canvas.getContext('2d'); ctx.clearRect(0, 0, 600, 450); ctx.drawImage(document.querySelector("video"), 0, 0); document.querySelector('img').src = canvas.toDataURL('image/png'); }
<div id="cubeOuter" class="cubeOuter"> <div class="cubeFace cubeFaceA"> 1 </div> <!-- other five faces go here --> </div>
.cubeFace { border: 2px solid black; border-radius: 5px; height: 100%; width: 100%; position: absolute; left: 0px; top: 0px; } .cubeFaceA { background: rgba(255, 0, 0, 0.5); } /* Other five styles go here. */
.cubeFaceA { -webkit-transform: rotateY(0deg); } .cubeFaceB { -webkit-transform: rotateY(-90deg); } .cubeFaceC { -webkit-transform: rotateY(180deg); } .cubeFaceD { -webkit-transform: rotateY(90deg); } .cubeFaceE { -webkit-transform: rotateX(90deg); } .cubeFaceF { -webkit-transform: rotateX(-90deg); }
.cubeOuter { -webkit-perspective: 1200; -webkit-perspective-origin: 300px 200px; -webkit-transition: -webkit-transform 2s; } .cubeFaceA { -webkit-transform: rotateY(0deg) translateZ(150px); } .cubeFaceB { -webkit-transform: rotateY(-90deg) translateZ(150px); } .cubeFaceC { -webkit-transform: rotateY(180deg) translateZ(150px); } .cubeFaceD { -webkit-transform: rotateY(90deg) translateZ(150px); } .cubeFaceE { -webkit-transform: rotateX(90deg) translateZ(150px); } .cubeFaceF { -webkit-transform: rotateY(-90deg) translateZ(150px); }
var rotateX = 0, rotateY = 0, rotateZ = 0, diff = 90; function rotateCube(e) { switch(e.keyCode) { case 49: rotateY -= diff; break; case 50: rotateY += diff; break; case 51: rotateX += diff; break; case 52: rotateX -= diff; break; case 53: rotateZ += diff; break; case 54: rotateZ -= diff; break; } var rX = "rotateX(" + rotateX + "deg) "; var rY = "rotateY(" + rotateY + "deg) "; var rZ = "rotateZ(" + rotateZ + "deg) "; $("cubeOuter").style.webkitTransform = rX + rY + rZ; } document.addEventListener('keypress', rotateCube, false);
navigator.geolocation.getCurrentPosition( function(position) { // Success handler. var acc = position.coords.accuracy; var lat = position.coords.latitude; var log = position.coords.longitude; var geoMap = document.getElementById("mapDiv"); var map = new google.maps.Map(geoMap, { }); var latLng = new google.maps.LatLng(lat, log); new google.maps.Marker({position: latLng, map: map}); map.setCenter(latLng); map.setZoom(15); }, function(evt) { // Error handler. } );
window.addEventListener('deviceorientation', function(event) { var alpha = event.alpha; // direction. var beta = event.beta, // front-to-back tit. var gamma = event.gamma; // left-to-right tilt. var layers = document.querySelectorAll('.layer'); for (var i = 0, zindex = 1, elem; elem = layers[i]; ++i, ++zindex) { var x = Math.round(1.5 * gamma * zindex); var y = Math.round(1.5 * beta * zindex); elem.style.left = x.toString() + 'px'; elem.style.top = y.toString() + 'px'; elem.style.webkitTransform = 'rotateY(' + (-2.0 * gamma) + 'deg) rotateX(' + (-2.0 * beta) + 'deg)'; } }, false);
@-webkit-keyframes rotate { from { -webkit-transform: rotateY(0deg); } to { -webkit-transform: rotateY(-360deg); } } .picture { -webkit-animation: rotate 6s linear 0s infinite normal; -webkit-transform-origin: 0px 0px; padding-left: 50px; border: 1px solid #ccc; background: rgba(255,255,255,0.2); box-shadow: inset 0 0 20px rgba(0,0,0,0.3); }
<div id="div1" ondrop="drop(event)" ondragover="allowDrop(event);" /> <img src="images/sky.jpg" draggable="true" ondragstart="drag(event);" />
function allowDrop(ev) { ev.preventDefault(); } function drag(ev) { ev.dataTransfer.setData("Text",ev.target.id); } function drop(ev) { ev.preventDefault(); var data=ev.dataTransfer.getData("Text"); ev.target.appendChild(document.getElementById(data)); }
var photoGroup = document.querySelector(".photoGroup"); setInterval(function () { if (navigator.onLine) { wheelElem.style.webkitAnimationPlayState = "running"; } else { wheelElem.style.webkitAnimationPlayState = "paused"; } }, 250);
function saveState(ev) { var imageNodes = document.querySelectorAll(".photoGroup img"); var imageIds = getCurrentImageIds(); var url = window.location.pathname + "#d=" + imageIds; history.pushState(null, null, url); } window.addEventListener('popstate', function(e) { var d = window.location.hash.split("=")[1]; setImages(d); });
<canvas id="canvas" width="800" height="500"></canvas>
function initCanvasDemo() { ctx = $('canvas').getContext("2d"); } function circle(x, y, r, color) { ctx.beginPath(); ctx.arc(x, y, r, 0, Math.PI*2, true); ctx.closePath(); ctx.fillStyle = color; ctx.fill(); } function rect(x, y, w, h, color) { ctx.beginPath(); ctx.rect(x,y,w,h); ctx.closePath(); ctx.fillStyle = color; ctx.fill(); }
function draw() { clear("#000000"); circle(x, y, 10); if (x + dx > canvasWidth || x + dx < 0) dx = -dx; if (y + dy > canvasHeight || y + dy < 0) dy = -dy; x += dx; y += dy; } function clear(backColor) { ctx.fillStyle = backColor; ctx.clearRect(0, 0, canvasWidth, canvasHeight); ctx.beginPath(); ctx.rect(0,0,canvasWidth,canvasHeight); ctx.closePath(); ctx.fill(); } setInterval(draw, 10);
function draw() { clear("#000000"); circle(x, y, 10); if (x + dx > canvasWidth || x + dx < 0) dx = -dx; if (y + dy > canvasHeight || y + dy < 0) dy = -dy; // Bounce off the ball only on colliding with the handle. if (x > handleX && x < handleX + handleW) dy = -dy; else clearInterval(intervalId); x += dx; y += dy; } function onMouseMove(evt) { if (evt.pageX > canvasMinX && evt.pageX < canvasMaxX) { handleX = evt.pageX - canvasMinX; } } document.addEventListener("mousemove", onMouseMove, false);
var header = new Uint8Array([ 0x52,0x49,0x46,0x46, // "RIFF" 0, 0, 0, 0, // put total size here 0x57,0x41,0x56,0x45, // "WAVE" 0x66,0x6d,0x74,0x20, // "fmt " 16,0,0,0, // size of the following 1, 0, // PCM format 1, 0, // Mono: 1 channel 0x44,0xAC,0,0, // 44,100 samples per second 0x88,0x58,0x01,0, // byte rate: two bytes per sample 2, 0, // aligned on every two bytes 16, 0, // 16 bits per sample 0x64,0x61,0x74,0x61, // "data" 0, 0, 0, 0 // put number of samples here ]).buffer; // Note: we just want the ArrayBuffer.
function makeWave(samples) { var bb = new WebKitBlobBuilder(); var dv = new DataView(header); dv.setInt32(4, 36 + samples.length, true); dv.setInt32(40, samples.length, true); bb.append(header); bb.append(samples.buffer); return bb.getBlob('audio/wav'); }
function playNote(frequency, duration) { var samplespercycle = 44100 / frequency; var samples = new Uint16Array(44100 * duration); var da = 2 * Math.PI / samplespercycle; for (var i = 0, a = 0; i < samples.length; i++, a += da) { samples[i] = Math.floor(Math.sin(a) * 32768); } var blob = makeWave(samples); var url = window.webkitURL.createObjectURL(blob); var player = new Audio(url); player.play(); player.addEventListener('ended', function(e) {window.webkitURL.revokeObjectURL(url);}, false); }Frequency: URL:
// Getting handle to the file system. window.requestFileSystem(window.TEMPORARY, 5*1024*1024 /*5MB*/, function (fs) { ... }, errorHandler); // Creating a file. fs.root.getFile('log.txt', {create: true, exclusive: true}, function(fileEntry) { .. }, errorHandler); // Reading a file. fileEntry.file(function(file) { ...}, errorHandler}; // Writing or appending to a file. fileEntry.createWriter(function(fileWriter) { ....}, errorHandler); // Removing a file. fileEntry.remove(function() { ..}, errorHandler);